JS-Plugin Pwstrength
HTML
JavaScript
CSS
Demo
<!DOCTYPE html> <html> <head> 	<title>Passwortsicherheit</title> 	<link rel="stylesheet" type="text/css" href="pwstrength.css" media="screen"> 	<script src="pwstrength.js"></script> 	<script type="text/javascript" language="JavaScript"> 		window.onload = function() { 			var pws = new Pwstrength({idpwelement:'pwort1',pwminlength:12,animtime:100}); 			pwort1.focus(); 		} 	</script> </head> <body> 	<div class="formdiv"> 		<h3><br>Prüfung der Passwortsicherheit</h3> 		<form action="login.php" method="post"> 			<p>Passwort:</p> 			<div id="password"> 				<input id="pwort1" class="pw" type="password" name="pwort1" value="" maxlength="20"> 				<div id="strenghtbar"><div id="bar" class="bar0"></div></div> 			</div> 		</form> 	</div> </body> </html>
/* RaWi-JS-Plugin:	Pwstrength 	*	JS-File:					pwstrength.js 	*	CSS-File:				pwstrength.min.css */ (function() { 	// Constructor 	this.Pwstrength = function(element) { 		// Globale Plugin-Element-Referenz 		this.pwstrength = null; 		// Bar-Element 		this.strenghtbar = null; 		// Option defaults 		var defaults = { 			idpwelement: 'pwort1', 			idstrenghtbar: 'bar', 			barclassname: 'bar', 			pwminlength: 12, 			animtime: 300 		} 		// Option defaults einlesen 		if (arguments[0] && typeof arguments[0] === "object") { 			this.options = extendDefaults(defaults, arguments[0]); 		} 		// Vars 		this.pstrength = 0; 		this.aktstrength = this.pstrength; 		this.passwort = ""; 		this.breite = 0; 		// Plugin-Elemente 		this.pwstrength = document.getElementById(this.options.idpwelement); 		this.strenghtbar = document.getElementById(this.options.idstrenghtbar); 		// EventListner 		this.pwstrength.addEventListener('keyup', this.handleKeyUp.bind(this)); 	} 	// --------------- 	// Plugin-Methoden 	// --------------- 	// Breite von strenghtbar animieren 	Pwstrength.prototype.animate = function (barelement,breite) { 		var schritte = 100; 		var step = 20 / schritte; 		var aktstep = 0; 		var steptime = this.options.animtime / schritte; 		var aktbreite = 20 * this.aktstrength; 		var baranimation = setInterval(function(){ animate() }, steptime); 		function animate() { 			if (aktstep > schritte) { 				clearInterval(baranimation); 			} else { 				aktstep += 1; 				if (breite > aktbreite) { 					elembreite = aktbreite + parseFloat( aktstep * step ) + '%'; 				} else { 					elembreite = (aktbreite - parseFloat( aktstep * step )) + '%'; 				} 				barelement.style.width = elembreite; 			} 		} 	} 	// KeyUp-Handler 	Pwstrength.prototype.handleKeyUp = function (event) { 		// Passwort-Strength auf 0 setzen 		this.pstrength = 0; 		// Passwort aus dem Eingabefeld holen 		this.passwort = this.pwstrength.value; 		// Prüfung Großbuchstaben 		if (this.passwort.match(/[A-ZÄÖÜ]{1,}/)) this.pstrength += 1; 		// Prüfung Kleinbuchstaben 		if (this.passwort.match(/[a-zäöüß]{1,}/)) this.pstrength += 1; 		// Prüfung Zahlen 		if (this.passwort.match(/\d{1,}/)) this.pstrength += 1; 		// Prüfung Sonderzeichen 		if (this.passwort.match(/[!@#$%^&*?_~].*?[!@#$%^&*?_~]/) 			 || this.passwort.match(/[!"@#\()+-.,;:§$%^&*?_~]/)) this.pstrength += 1; 		// Prüfung Länge 		if (this.passwort.length >= this.options.pwminlength) this.pstrength += 1; 		// Bar-Class bestimmen 		this.strenghtbar.className = this.options.barclassname + this.pstrength; 		// Bar animieren wenn aktstrength <> pstrength 		if (this.aktstrength != this.pstrength) { 			// Berechnung der Breite in Prozent 			this.breite = 20 * this.pstrength; 			// Bar animieren 			this.animate(this.strenghtbar,this.breite); 			// aktstrength aktualisieren 			this.aktstrength = this.pstrength; 		} 	} 	// ------------------------------ 	// Option defaults implementieren 	// ------------------------------ 	function extendDefaults(source, properties) { 		var property; 		for (property in properties) { 			if (properties.hasOwnProperty(property)) { 				source[property] = properties[property]; 			} 		} 		return source; 	} }());
/* Styles Plugin Pwstrength */ * { box-sizing:border-box; } .formdiv { width:300px; margin:0 auto; } .pw { 	height:27px; 	width:300px; 	font-size:15px; 	text-align:left; 	color:#000; 	background:#eee; 	outline:none; 	border:1px solid #ccc; 	padding:4px 4px 4px 4px; 	margin:5px 0px 10px 0px; } #strenghtbar { 	height:3px; 	width:100%; 	position:relative; 	background:#ccc; 	top:-10px; 	display:block; } .bar0 { height:3px; width:100%; background:#ccc; } .bar1 { height:3px; width:0%; background:#cc0000; position:absolute; left:0px; } .bar2 { height:3px; width:0%; background:#fd8f00; position:absolute; left:0px; } .bar3 { height:3px; width:0%; background:#fdd600; position:absolute; left:0px; } .bar4 { height:3px; width:0%; background:#fdee00; position:absolute; left:0px; } .bar5 { height:3px; width:0%; background:#00aa00; position:absolute; left:0px; }
JS-Plugin zur Prüfung der Passwortsicherheit
Das Passwort ist nur sicher, wenn es Groß- und Kleinbuchstaben, Zahlen und Sonderzeichen enthält und eine vorgegebene Mindestlänge besitzt.
Passwort:
Dieses Plugin visualisiert die Sicherheit eines Passworts.
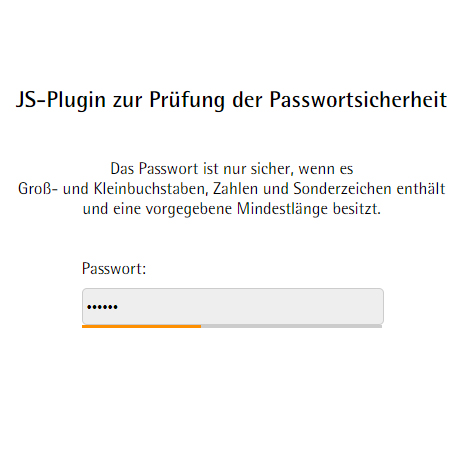
Sorry, aber die Darstellung der Codes dieses Beispiels ist auf kleinen Displays zu unübersichtlich.Sie können sich jedoch die Codes downloaden und das Plugin auf ihrem PC/Laptop/Tablet ausprobieren.